Scroll Transform
A versatile UI element that provides a flexible and customizable way to organize and display menu items within your application.
Usage
Apple style dock
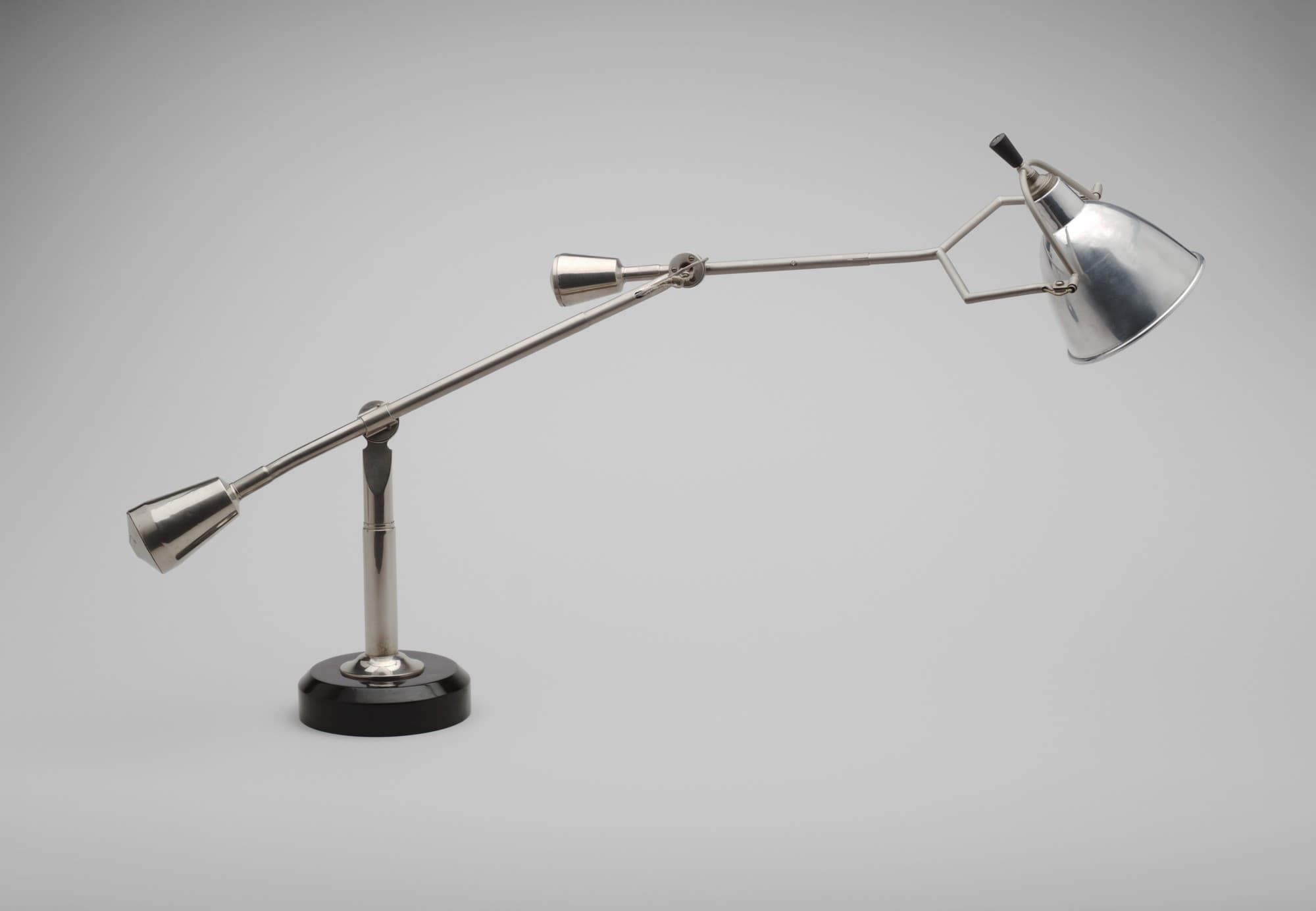
Code
'use client';
import React, { useState, useEffect, useRef } from 'react';
import { motion, useScroll, useTransform } from 'framer-motion';
import Image from 'next/image';
export default function ScrollTransform({
children,
}: {
children: React.ReactNode;
}) {
const container = useRef(null);
const { scrollYProgress } = useScroll({
target: container,
offset: ['start end', 'end start'],
});
const y = useTransform(scrollYProgress, [0, 1], ['-6%', '6%']);
return (
<div ref={container} className='relative size-full'>
<motion.div className='relative size-full' style={{ y }}>
{children}
</motion.div>
</div>
);
}
Component API
ScrollTransform
Prop | Type | Default | Description |
---|---|---|---|
children | ReactNode | The content to display in the button. | |
asChild | boolean | false | Replaces the button tag with the provided child. |
icon | ReactElement | <ArrowBigRight size={28} /> | Colors for the waves that animate on hover. |
prependIcon | boolean | false | The visual style of the button. |
size | 'default' | 'large' | 'default' | The size of the button, with 'large' being bigger. |
ref | Ref<Button or Link Element> | Provides a reference to the button or link DOM element, depending on asChild . |